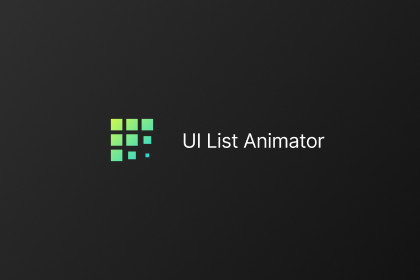
UIListAnimation is a powerful Unity UI system for smooth list/grid animations. Supports position, scale, rotation and alpha effects. Perfect for game menus and easy to set up.Dependencies:DOTween/Pro required (v1.2.0+)Unity 2020.3 or higherTested on Unity 2020.3+Notes:CanvasGroup needed for alpha animationsConfigure animations in Unity Editor recommendedDemo scenes included for quick startFull documentation included in packageSupport:Email: 455471553@qq.comUse document links:https://jxaytl13.github.ioUIListAnimation is a flexible animation system for Unity UI elements, designed to create smooth and professional list/grid animations. It offers:Multiple animation types (position, scale, rotation, alpha)Two layout modes (normal list and grid)Visual editor for easy setupCustom timing and easing controlsRuntime API supportPerfect for:Game menusInventory systemsAchievement panelsShop interfacesAny scrolling UI contentFeatures:Easy to configure with visual editorHighly customizable animationsOptimized performanceComprehensive documentationDemo scenes includedRequires DOTween and Unity 2020.3+Use document links: https://jxaytl13.github.ioUIListAnimation API DocumentationTable of Contents1. [Introduction](#introduction)2. [System Requirements](#system-requirements)3. [Installation](#installation)4. [Core Components](#core-components)5. [API Reference](#api-reference)6. [Code Examples](#code-examples)7. [Best Practices](#best-practices)IntroductionUIListAnimation is a powerful and flexible Unity UI list animation system that makes it easy to add beautiful animation effects to UI elements in lists or grids. This animation system depends on DOTween.System RequirementsUnity 2020.3 or higherDOTween/Pro (required)InstallationImport the UIListAnimationSystem folder into your Unity projectEnsure DOTween/Pro plugin is installedCore ComponentsUIListAnimatorThe main animation control component responsible for managing and playing UI list animations.AnimationConfigAnimation configuration file used to store animation properties and settings.API ReferenceUIListAnimator ClassProperties| Property Name | Type | Description ||--------------|------|-------------|| Config | AnimationConfig | Animation configuration file || PlayOnEnable | bool | Whether to automatically play animation when enabled || AdditionalDelay | float | Additional delay time || PlayMode | PlayMode | Play mode (Normal/Grid) |MethodsPlay()Start playing the animation.```csharppublic void Play()```Stop()Stop the current playing animation.```csharppublic void Stop()```AnimationConfig ClassBasic Settings| Property | Type | Description ||----------|------|-------------|| StartDelay | float | Delay time before animation starts || ItemMode | ItemMode | Time mode (Interval/Duration) || ItemInterval | float | Time interval between items (Interval mode) || ItemDuration | float | Animation duration for items (Duration mode) |Animation Property ConfigurationEach animation property can include:| Property | Type | Description ||----------|------|-------------|| Type | AnimationType | Animation type (Position/Scale/Rotation/CanvasGroup) || StartValue | Vector3/float | Start value || EndValue | Vector3/float | End value || Duration | float | Animation duration || EaseType | Ease | Easing type || CustomCurve | AnimationCurve | Custom animation curve |Code ExamplesBasic Usage```csharp// Get UIListAnimator component and play animationvar animator = gameObject.GetComponent();animator.Play();// Set configuration and playvar animator = gameObject.GetComponent();var config = Resources.Load("Path/To/Your/Config");animator.Config = config;animator.Play();// Set delay and playvar animator = gameObject.GetComponent();animator.AdditionalDelay = 0.5f;animator.Play();// Stop animationanimator.Stop();```Best PracticesRecommended UI Structure```- List Container (UIListAnimator) - Item 1 - Item Parent <-- Target node for animation (requires CanvasGroup component) - Content1 - Content2 ... - Item 2 - Item Parent <-- Target node for animation (requires CanvasGroup component) - Content1 - Content2 ...```Performance Optimization TipsSet reasonable animation duration and intervalsAvoid too many simultaneous animationsUse object pooling for optimization when appropriateImportant NotesCanvasGroup animation type requires CanvasGroup component on target objects. If not, it will be automatically mounted.Grid size modifications in editor will automatically adjust sequence numbersPreview animation button is only available during runtime`Play()` method can be called repeatedly, it will automatically stop previous animation and start new oneEnsure a valid animation configuration is set before calling `Play()`