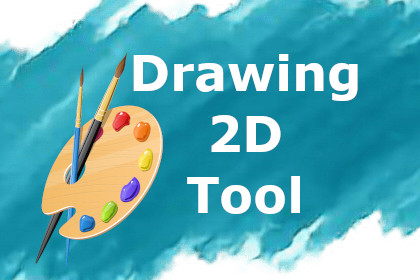
2D Drawing System - Technical GuideThe 2D Drawing System allows users to draw freely on a sprite in Unity. This package enables drawing with a brush, adjusting brush size and color, and clearing the canvas. It's ideal for 2D games or applications where dynamic drawing is needed. Below are the features and technical details of the system.Main Features:Users can dynamically change the brush color and size during drawing, allowing for customizations based on their needs.Brush Color and Size Adjustments:Users can draw freely by dragging the mouse. The system supports drawing lines and free-form shapes.Freehand Drawing:The drawing is performed on a Texture2D object, which is then converted to a Sprite and displayed in the scene.Sprite-Based Drawing:The canvas can be cleared by resetting the sprite to its background color.Clear the Drawing:Technical Features:Brush Settings:Brush Size: The brushSize variable controls the size of the brush in pixels.Brush Color: The brushColor variable allows you to select the drawing color.Drawing Method:Mouse Drag Drawing: Users draw by dragging the mouse. The mouse coordinates are transformed into pixel coordinates on the sprite, where the drawing is applied.Bresenham Line Algorithm: The Bresenham algorithm is used for drawing straight lines between two points, ensuring smooth and accurate line drawing.Sprite and Texture Management:Texture2D: Drawings are made on a Texture2D, which is then converted to a sprite to be rendered in the scene.Sprite Updates: After each drawing action, the texture is updated with drawingTexture.Apply(), ensuring the drawing is immediately visible.Dynamic Sprite Creation: A Texture2D is created, converted to a sprite, and assigned to a SpriteRenderer for display.Drawing Reset:ClearSprite Method: The ClearSprite() method clears all drawings by resetting the sprite to the background color.Events and User Interaction:OnMouseDrag: Drawing happens while dragging the mouse, using the OnMouseDrag method.OnMouseDown and OnMouseUp: Drawing starts on mouse down and stops on mouse up.Usage Instructions:Setup:Attach this script to any GameObject that should support drawing. The script automatically adds SpriteRenderer and PolygonCollider2D components if they don't exist.The drawing functionality works immediately once the script is added to the GameObject.Brush Color and Size:Use the SetColor() method to change the brush color.Use the SetSize() method to change the brush size.Drawing:To draw, simply click and drag the mouse over the sprite. The OnMouseDrag method will track the mouse movement and update the drawing accordingly.Clearing the Canvas:To clear the drawing, call the ClearSprite() method. This will reset the sprite to the background color.Customization:The brush size and color can be adjusted at any time using the provided methods. Additionally, the background color can be set when initializing the system.Code Snippets for Usage:Here are some basic examples of how to use the methods provided:csharpKopyalaDüzenle// Change brush color to reddrawingTool.SetColor(Color.red);// Change brush size to 10drawingTool.SetSize(10);// Clear the canvasdrawingTool.ClearSprite();Additional Information:The package is designed to be easy to integrate into any 2D Unity project.It uses simple Unity components like SpriteRenderer and PolygonCollider2D, making it compatible with most 2D projects.For optimal performance, the system uses Texture2D to store the drawings and applies updates only when necessary.